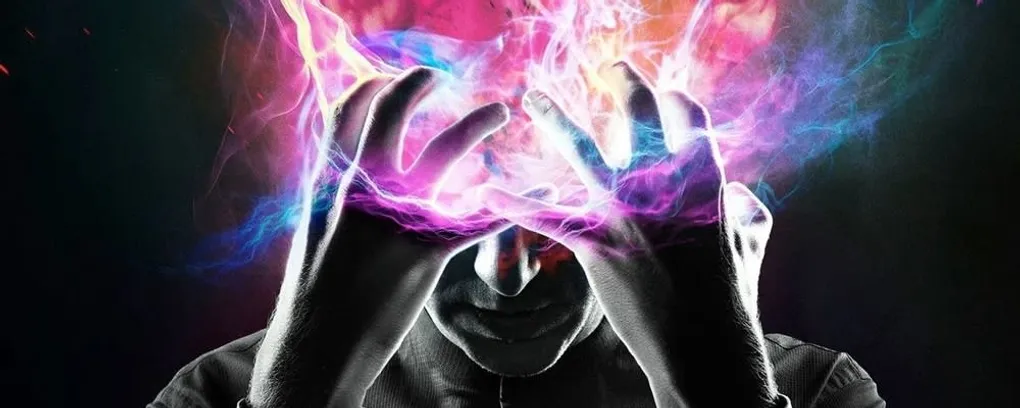
Jestip, clear mocks automatically
Let’s take the following code as example.
function functionYouNeedToMock(a, b) {
return a + b
}
beforeEach(() => {
jest.clearAllMocks()
})
functionYouNeedToMock = jest.fn()
it("should call function", () => {
functionYouNeedToMock()
expect(functionYouNeedToMock).toBeCalledTimes(1)
})
it("should call function", () => {
functionYouNeedToMock()
expect(functionYouNeedToMock).toBeCalledTimes(1)
})
It considers that you mocked a function and you want your tests to be independent. So you’re using the jest.clearAllMocks()
function to avoid undesired behaviours.
Did you know that you can activate the clearMocks
option in the config in order to avoid calling jest.clearAllMocks()
in each test suite ?
// jest.config.js
module.exports = {
clearMocks: true,
}
Now you can write
function functionYouNeedToMock(a, b) {
return a + b
}
functionYouNeedToMock = jest.fn()
it("should call function", () => {
functionYouNeedToMock()
expect(functionYouNeedToMock).toBeCalledTimes(1)
})
it("should call function", () => {
functionYouNeedToMock()
expect(functionYouNeedToMock).toBeCalledTimes(1)
})
Thanks for reading